IMS DB Program Coding
An application program that includes DL/I calls cannot run on its own; it requires a JCL to initiate the IMS DL/I batch module. The batch initialization module in IMS is called DFSRRC00. The application program and the DL/I module work together during execution. The following diagram explains the structure of an application program that utilizes DL/I calls to access a database.
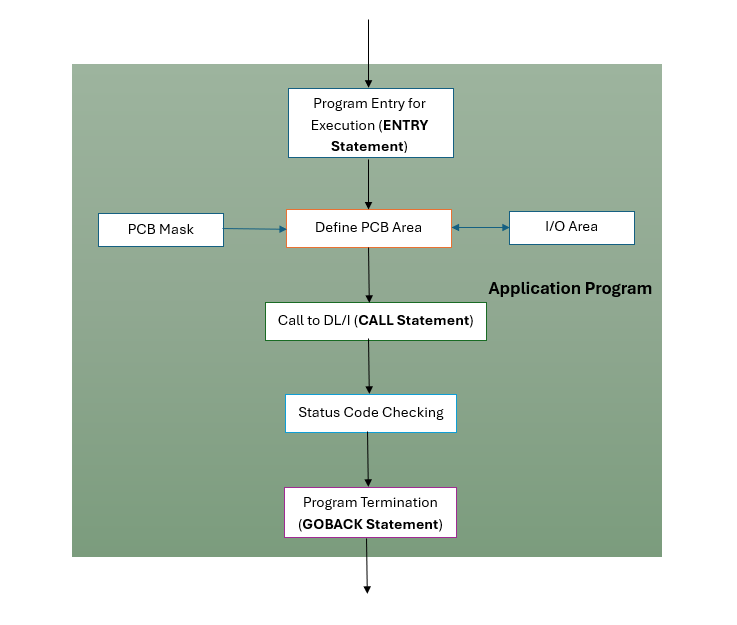
IMS uses DL/I as its interface for application programs to access and manipulate data. Programming in IMS involves writing application programs, typically in COBOL, that make DL/I calls to perform operations like reading, inserting, updating, or deleting data in the IMS database.
Key Components in IMS DB Programming
An IMS application program interacts with the database through several key components:
- ENTRY Statement: This statement specifies that the program will use certain Program Communication Blocks (PCBs).
Example: In a COBOL program, you might have:
This indicates that the program will use the PCB named COMPANY-PCB-MASK.ENTRY 'DLITCBL' USING COMPANY-PCB-MASK.
- PCB Mask: This is a data structure in the program that corresponds to the PCB defined in the Program Specification Block (PSB).
It receives status codes and other information from IMS after each DL/I call.
Example: A PCB mask might look like:
01 COMPANY-PCB-MASK. 05 CMP-DBD-NAME PIC X(8). 05 CMP-SEGMENT-LEVEL PIC XX. 05 CMP-STATUS-CODE PIC XX. ...
- I/O Area: This is a working storage area in the program used to send data to and receive data from the IMS database.
Example:
01 SEGMENT-I-O-AREA PIC X(150).
- DL/I Calls: These are the actual calls made from the application program to IMS to perform operations.
Each call specifies a function code (like 'GU' for Get Unique), the PCB mask, the I/O area, and optionally, Segment Search Arguments (SSAs).
Example:
CALL 'CBLTDLI' USING DLI-GU COMPANY-PCB-MASK SEGMENT-I-O-AREA.
- Status Code Checking: After each DL/I call, IMS returns a status code in the PCB mask to indicate the success or failure of the operation. The program should check this code to handle errors appropriately.
- Program Termination: Instead of using 'STOP RUN', IMS programs use 'GOBACK' to return control to IMS,
allowing it to perform necessary cleanup operations.
Example:
GOBACK.
Example: Company-Project-Employee Structure
Consider a company database where we have segments for companies, projects, and employees. In COBOL, the segment layouts might be defined as:
01 COMPANY-SEGMENT.
05 COMPANY-ID PIC X(5).
05 COMPANY-NAME PIC A(30).
01 PROJECT-SEGMENT.
05 PROJECT-ID PIC X(5).
05 PROJECT-NAME PIC A(30).
05 COMPANY-ID PIC X(5).
01 EMPLOYEE-SEGMENT.
05 EMPLOYEE-ID PIC X(5).
05 EMPLOYEE-NAME PIC A(25).
05 PROJECT-ID PIC X(5).
In this structure:
- COMPANY-SEGMENT is the root segment containing company information.
- PROJECT-SEGMENT is a child of COMPANY-SEGMENT, containing project details.
- EMPLOYEE-SEGMENT is a child of PROJECT-SEGMENT, containing employee details.
An application program would use DL/I calls to interact with these segments, such as retrieving a company's information, listing its projects, or recording a new employee under a project.
IMS Services
The following IMS services are used by the application program −
- Accessing database records
- Issuing IMS commands
- Issuing IMS service calls
- Checkpoint calls
- Sync calls
- Sending or receiving messages from online user terminals